A colleague recently asked me how to access the Microsoft Graph API using PowerShell without specifying his user account or credentials. So here’s a little post about the required configuration to authenticate against the OAuth 2.0 endpoint of Azure AD with an app registration. This is especially useful for automation services like Azure automation.
At the end of this post you’ll find a PowerShell template.
Gather application information
Create a new client secret for your app and note down the following values:
- Client Secret
- Application ID (client ID)
- Directory ID (tenant ID)
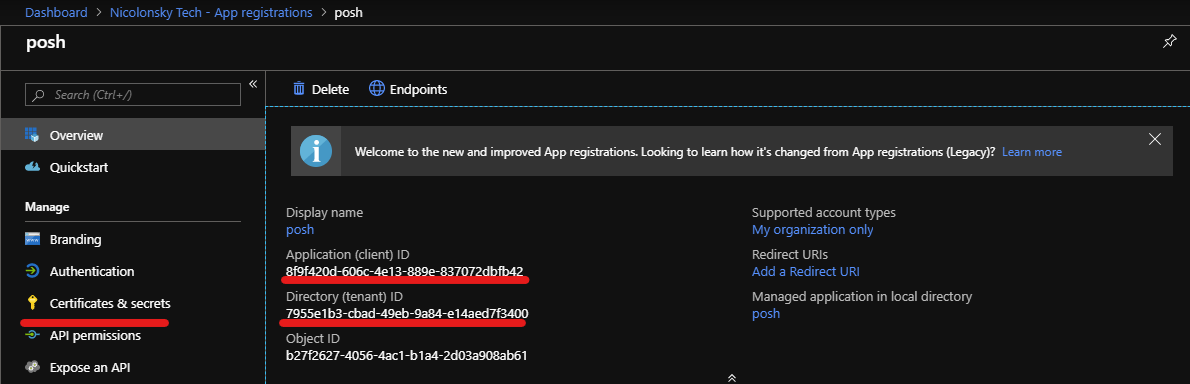
Request authentication token
In order to use the Graph API we need an authentication token. The information we gathered before is now sent to the Azure AD OAuth 2.0 endpoint.
|
|
In the request supply a body with the following content:
|
|
The scope and grant_type are required attributes. A full example looks like:
|
|
If everything works we receive an access token object as HTML 200/ok response:
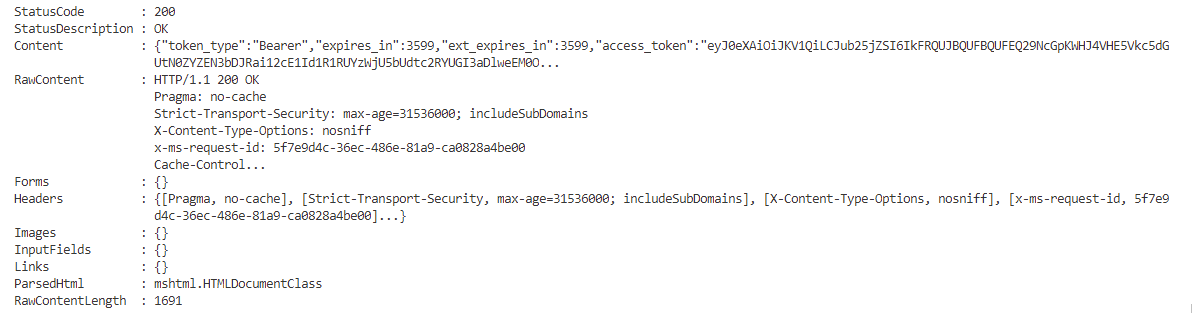
Using the access token
Now you’re ready to make requests to the Graph API. Just include the access token in your request’s header. Make sure that your application has the required permissions assigned and granted with admin consent!
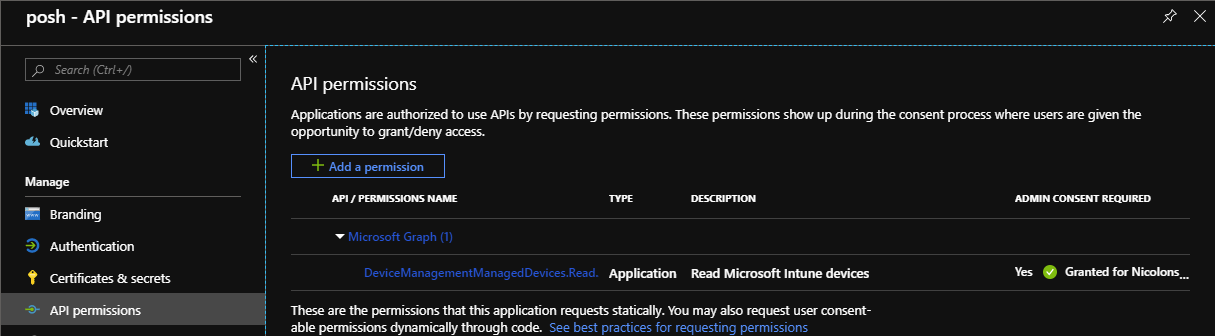
PowerShell template
Here’s a little PowerShell template which contains all the logic required to authenticate against the Graph API - hope it saves you some time:
- Reference: Microsoft Docs